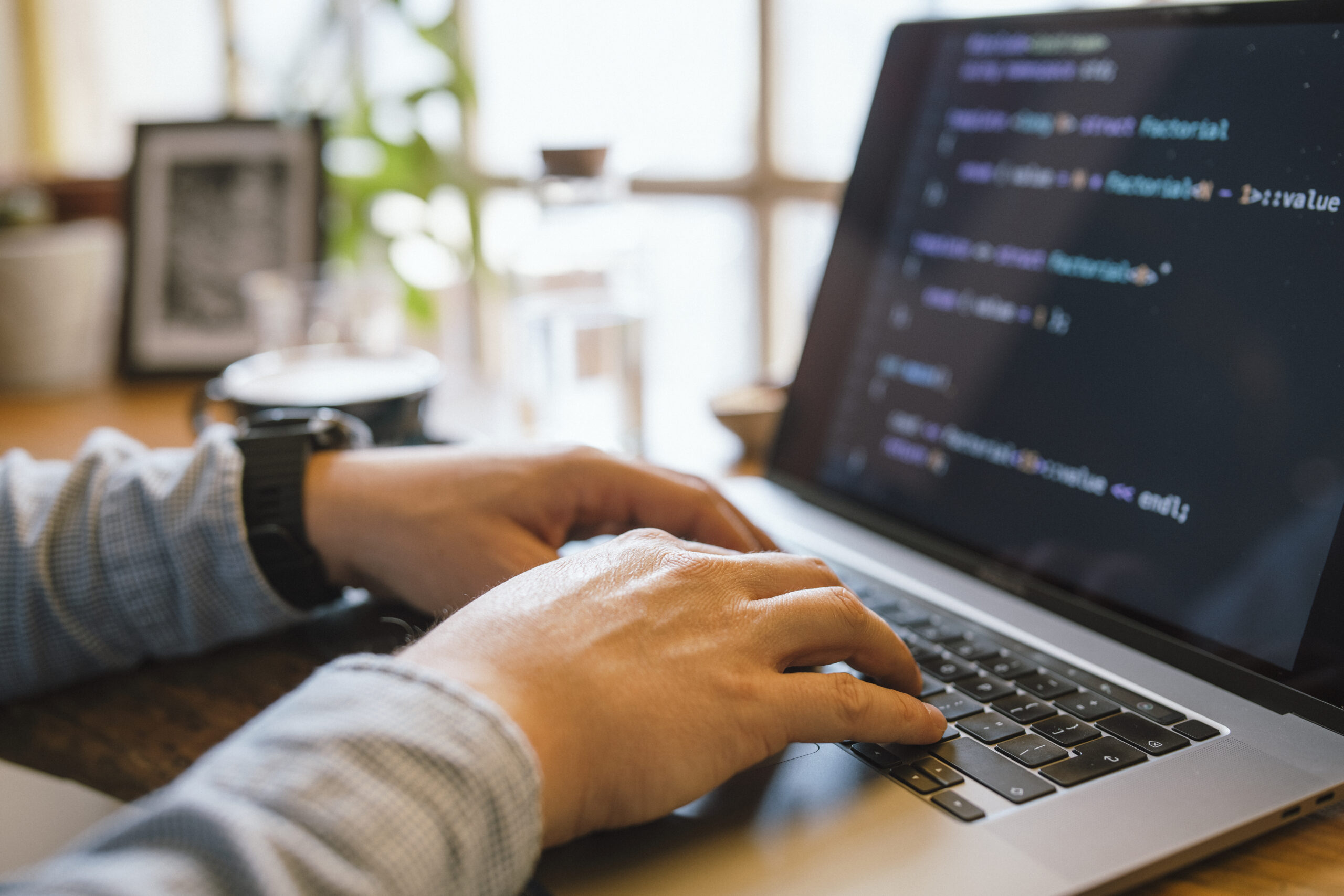
Debugging is One of the more important — nevertheless normally overlooked — expertise within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Feel methodically to resolve difficulties proficiently. No matter if you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially increase your productiveness. Listed below are numerous techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use daily. Whilst crafting code is a person Component of growth, realizing how you can connect with it properly in the course of execution is equally significant. Present day development environments occur Outfitted with effective debugging capabilities — but quite a few developers only scratch the surface of what these applications can perform.
Take, for example, an Built-in Development Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the value of variables at runtime, phase via code line by line, and perhaps modify code about the fly. When utilized the right way, they Enable you to observe particularly how your code behaves throughout execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI troubles into workable tasks.
For backend or program-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model Command methods like Git to grasp code heritage, obtain the exact moment bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your development atmosphere in order that when concerns come up, you’re not dropped at nighttime. The higher you recognize your instruments, the more time it is possible to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently missed — techniques in productive debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders need to have to create a consistent environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected sufficient information and facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, contemplate crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply assist expose the challenge but also avoid regressions Sooner or later.
Sometimes, The problem can be environment-distinct — it'd happen only on specific running units, browsers, or below certain configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, take a look at opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance right into a concrete problem — and that’s exactly where developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Lots of developers, especially when underneath time strain, look at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in Those people circumstances, it’s important to look at the context by which the error happened. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger concerns and supply hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout development, Facts for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice details as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments wherever stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers need to technique the procedure similar to a detective resolving a mystery. This state of mind will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the basis result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What might be triggering this conduct? Have any modifications lately been made into the codebase? Has this difficulty transpired just before below similar instances? The goal will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and Allow the effects direct you closer to the reality.
Shell out near attention to modest specifics. Bugs often disguise inside the the very least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race problem. Be thorough and affected individual, resisting the urge to patch The problem without thoroughly knowing it. Non permanent fixes could conceal the true problem, only for it to resurface afterwards.
And finally, keep notes on Whatever you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential challenges and assist Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical techniques, solution difficulties methodically, and come to be more effective at uncovering hidden difficulties in complex programs.
Generate Tests
Composing checks is among the most effective approaches to transform your debugging skills and All round growth performance. Checks not only aid catch bugs early and also function a security Web that offers you self-confidence when producing alterations on your codebase. A well-tested software is simpler to debug as it helps you to pinpoint accurately where and when a problem occurs.
Begin with unit assessments, which target particular person capabilities or modules. These smaller, isolated checks can immediately expose whether a selected bit of logic is working as envisioned. Any time a test fails, you immediately know where to appear, considerably decreasing time spent debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear right after previously becoming preset.
Upcoming, combine integration exams and finish-to-end checks into your workflow. These enable be sure that a variety of elements of your application do the job alongside one another efficiently. They’re specifically helpful for catching bugs that manifest in advanced techniques with multiple factors or expert services interacting. If one thing breaks, your tests can inform you which Portion of the pipeline unsuccessful and underneath what circumstances.
Crafting exams also forces you to definitely Consider critically about your code. To check a function thoroughly, you may need to know its inputs, envisioned outputs, and edge circumstances. This volume of knowing Normally sales opportunities to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In short, composing assessments turns debugging from a aggravating guessing video game into a structured and predictable method—supporting you capture extra bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Remedy immediately after Alternative. But one of the most underrated debugging resources is just stepping absent. Having breaks helps you reset your thoughts, minimize stress, and sometimes see The problem from the new point of view.
When you are also near to the code for also prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind becomes less economical at trouble-resolving. A brief walk, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious work in the history.
Breaks also support stop burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally stuck, is don't just unproductive but will also draining. Stepping away enables you to return with renewed Electrical power and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it really truly causes quicker and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Improper.
Start out by inquiring on your own a handful of key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or knowing and allow you to Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your development journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you deal with adds a fresh layer towards your skill established. So future time you squash a bug, take a minute get more info to replicate—you’ll come absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.